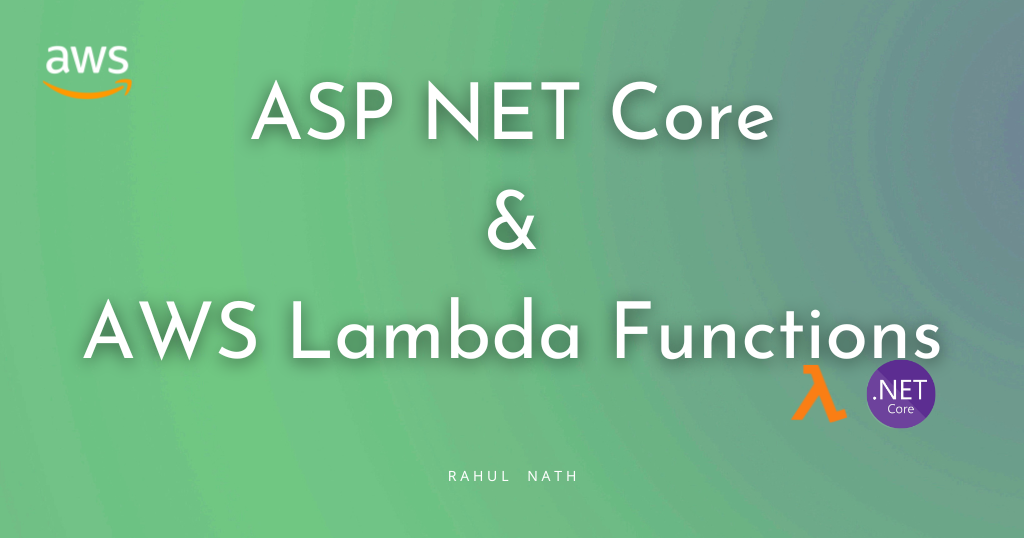
How To Build and Host ASP NET Core Applications on AWS Lambda Functions
Table of Contents
This article is sponsored by AWS and is part of my AWS Series.
AWS Lambda is a compute service that lets you run code without provisioning or managing servers.
You can build application APIs on Lambda Function with API Gateways (REST or HTTP API) or Function URL’s.
But what if you have existing APIs built using ASP NET Web API and want to use AWS Lambda Function?
You might not want to go through all the work to migrate them over to independent Lambda functions and build the API from scratch.
In this post, I will show you how you can easily host ASP Net Web APIs on Lambda Functions.
Setting up ASP NET Core on Lambda
You must add a reference to Amazon to enable Lambda Hosting for any ASP NET Core application.Lambda.AspNetCoreServer.Hosting Nuget package.
You can find the complete source code of the Nuget package here on Github.
Once added, enable Lambda Hosting using the extension method AddAWSLambdaHosting
as shown below.
builder.Services.AddAWSLambdaHosting(LambdaEventSource.RestApi);
Depending on how you plan to expose the ASP NET API, you can choose different options for the LambdaEventSource
. The supported values are RestApi
, HttpApi
and ApplicationLoadBalancer
as shown below.
public enum LambdaEventSource
{
//
// Summary:
// API Gateway REST API
RestApi,
//
// Summary:
// API Gateway HTTP API
HttpApi,
//
// Summary:
// ELB Application Load Balancer
ApplicationLoadBalancer
}
Deploy To AWS Lambda
You can use the .NET Core Global Tools for AWS to deploy an ASP NET application to Lambda from the command line.
Navigate to the root of the project folder for the ASP NET application and run the below command to deploy the application to Lambda.
dotnet lambda deploy-function
The CLI tool prompts for more inputs to configure the Lambda Function like the runtime (dotnet6), Function name, IAM Role, default timeout, etc.
Enter the relevant values required for your application, and it will successfully deploy to the Lambda environment.
I prefer to set up a build-deploy pipeline to deploy code in a real-world application. Check out the video below on how you can use CloudFormation templates from GitHub Actions to automatically deploy code changes to a Lambda Function.
Right Click Deploy From Visual Studio
When creating a Lambda Project, Visual Studio shows the option to deploy a Lambda Function right from within Visual Studio.
However, this option is not available for an ASP NET Application by default. To enable this option, you can edit the csproj
file and add in the AWSProjectType
node and set it to the value 'Lambda'.
<AWSProjectType>Lambda</AWSProjectType>
Once added, right-click on the project in Visual Studio Solution Explorer, and you will see the option Publish to AWS Lambda...
.
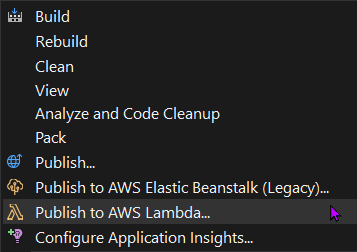
Use this and follow the GUI dialog to deploy the Lambda Function.
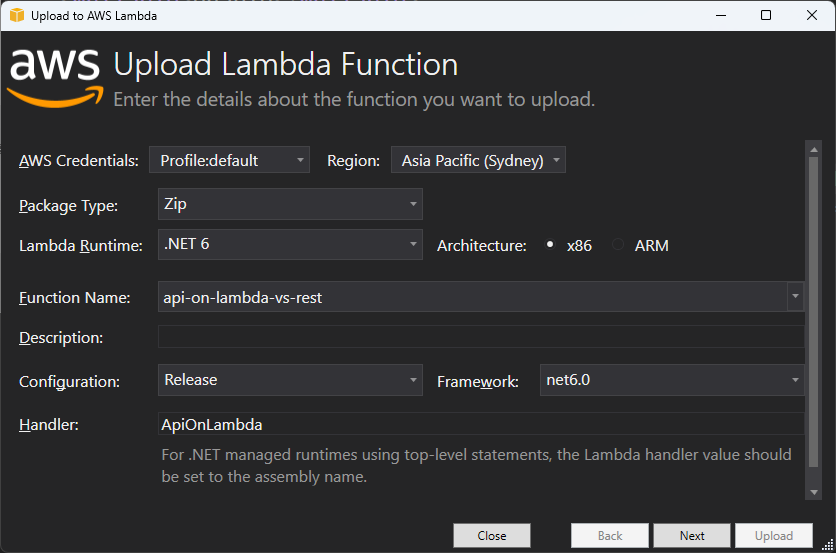
If you are new to AWS Lambda, I recommend checking out my Getting Started with AWS Lambda article below.
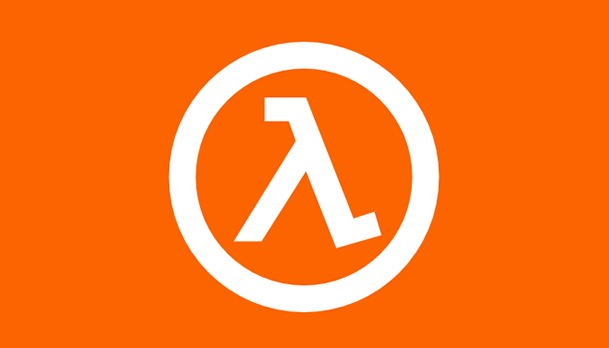
Exposing ASP NET API Over a URL
With the Lambda Function deployed with the ASP NET Web Application, we need to expose it over a URL for other applications to access.
There are different ways to achieve this, as shown below.
ASP NET API Over Lambda Function URL
A Function URL is a dedicated HTTP(S) endpoint for your Lambda function.
When enabling Function URLs, Lambda automatically generates a unique URL endpoint. Once you create a function URL, its URL endpoint never changes.
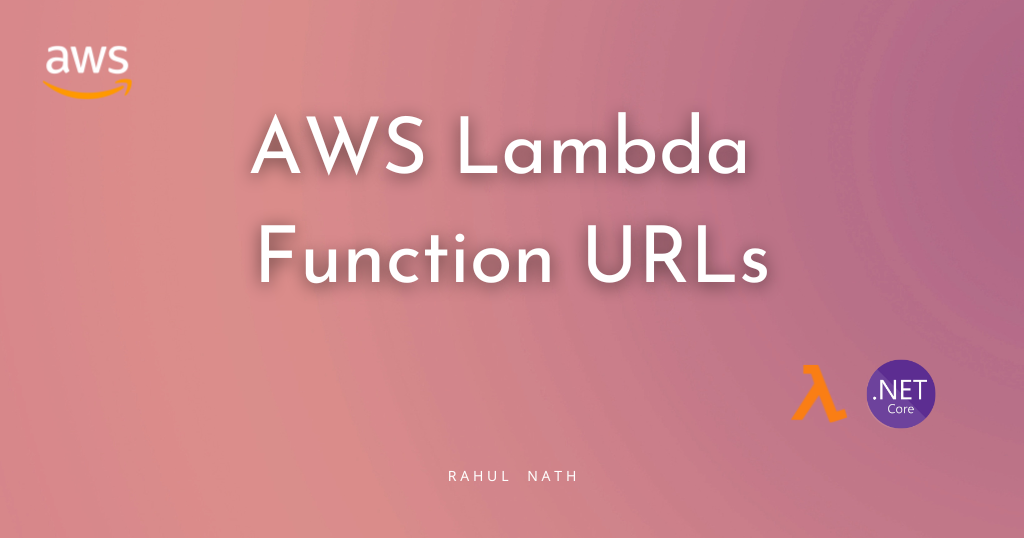
When using Function URLs to expose ASP NET Api on Lambda Function, use the LambdaEventSource
with the value HttpApi
.
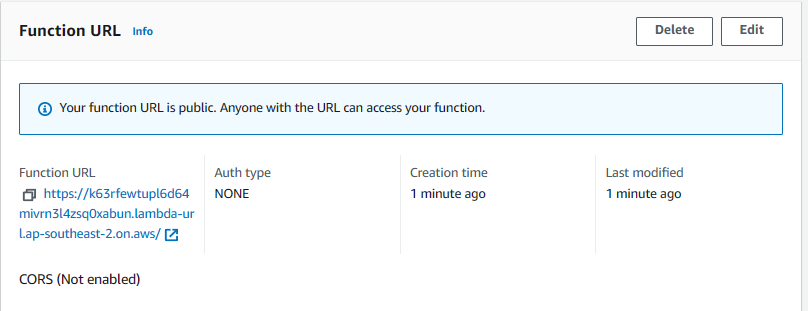
Navigate to the Function URL to access the ASP NET API Application. You can also add Function URL Auth to protect the API endpoint for specific applications or users with access.
ASP NET API Over AWS API Gateway
Amazon API Gateway is a fully managed service that helps developers build and maintain APIs at any scale. The Gateway is a ‘front door’ to your business data and backend services.
API Gateway is often mentioned along with Serverless technologies, especially AWS Lambda, since exposing the Function over an HTTP endpoint makes it very easy.
API Gateway supports two modes of creating APIs
HTTP APIs
HTTP APIs are the latest services designed for low-latency, cost-effective integrations with different AWS services. Compared to the previous generation REST APIs it has a lot of advantages. However, there are also certain missing features. So before diving in, ensure HTTP APIs are the right choice for you (which most of the time will be ).
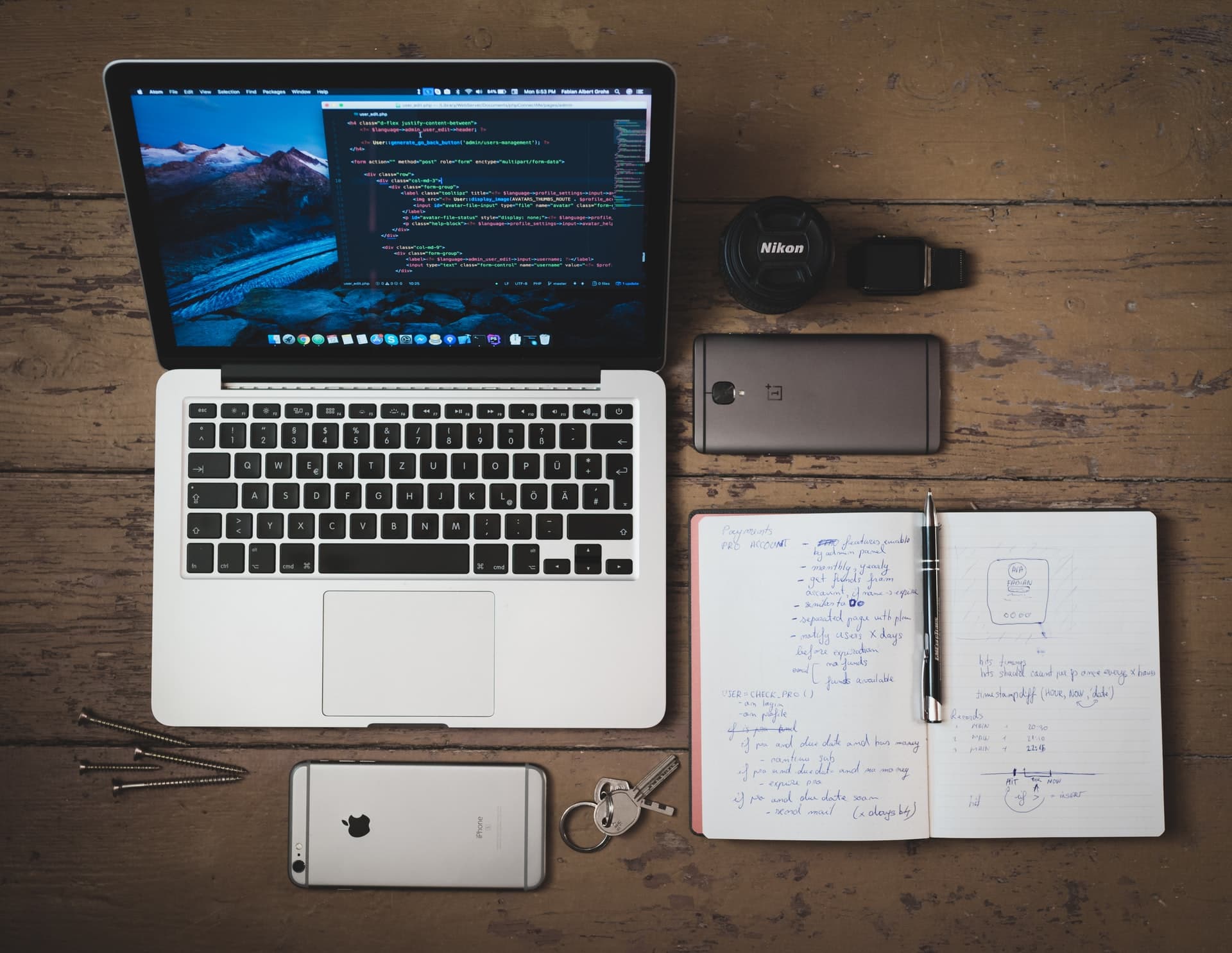
To expose as HTTP APIs, use the LambdaEventSource
with the value HttpApi
, same as with the Function URL.
REST APIs
While HTTP APIs are designed with minimal and cost-effective features, REST APIs provide more advanced features such as API keys, per-client throttling, request validation, private API endpoints, etc.
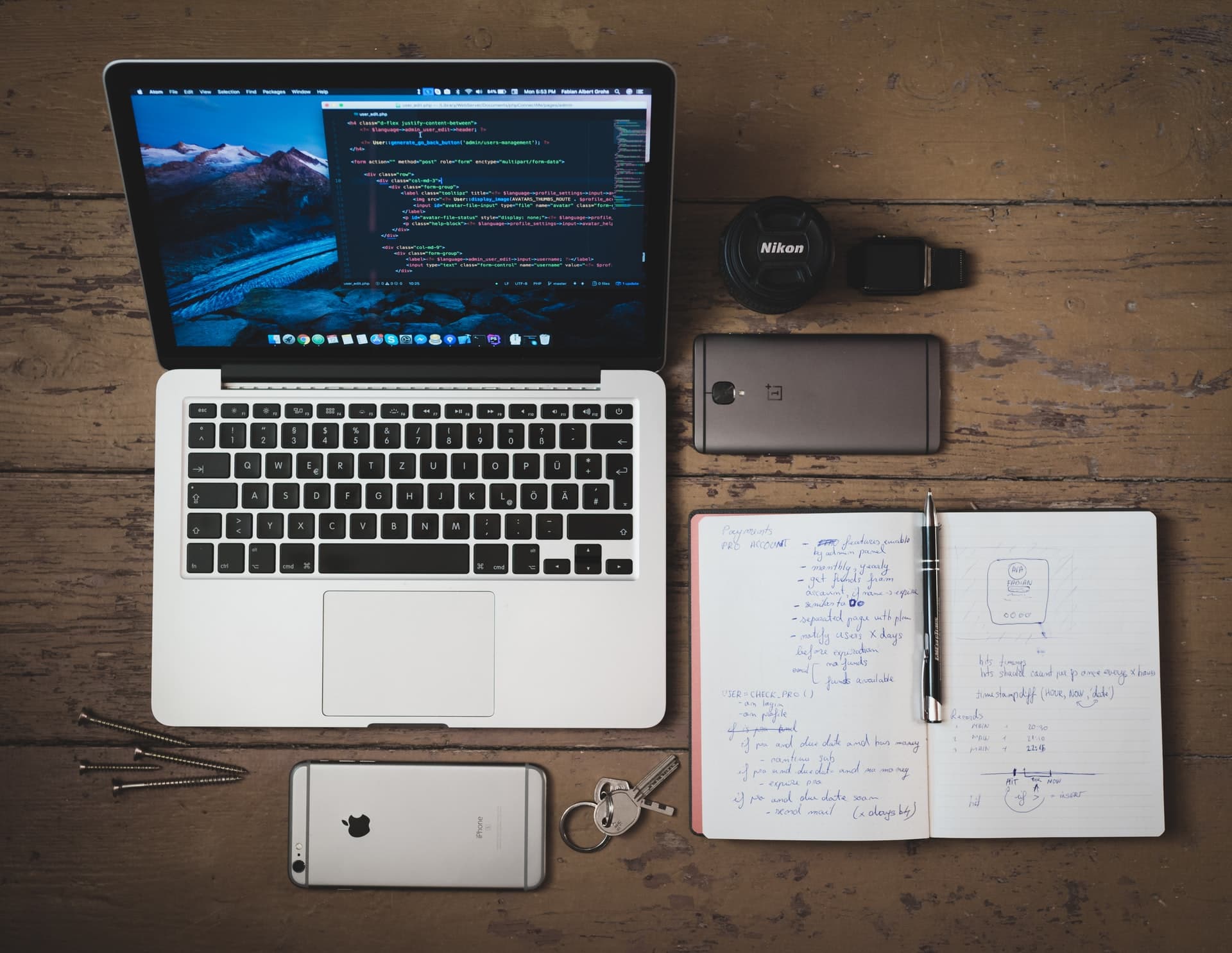
When exposing Lambda Functions over API Gateway REST APIs, set the LambdaEventSource
as RestApi
.
I hope this helps you to build and run your ASP NET Applications on AWS Lambda Function.
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.