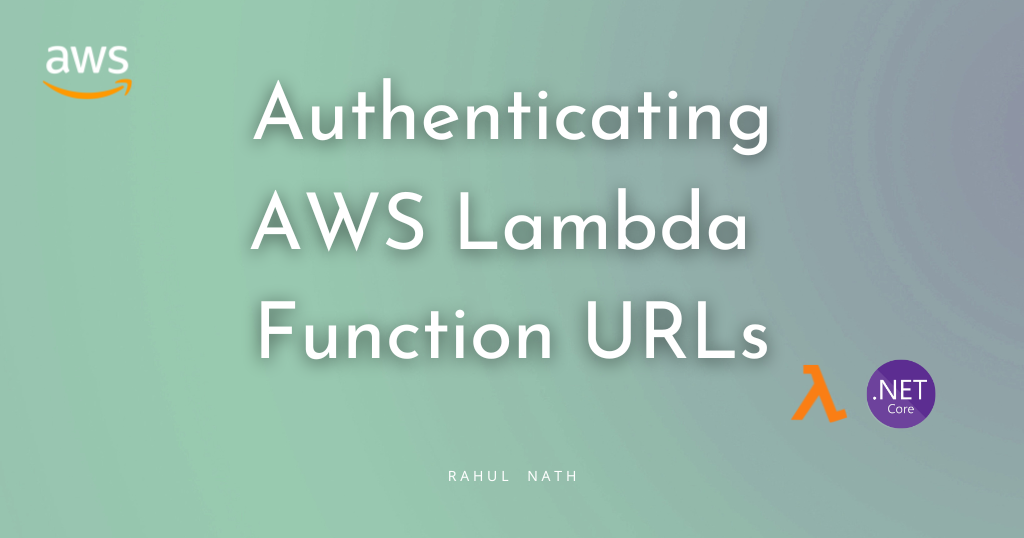
How To Secure and Authenticate AWS Lambda Function URLs
Table of Contents
This article is sponsored by AWS and is part of my AWS Series.
In a previous blog post, Function URLs - Quick and Easy way to Invoke AWS Lambda Functions over HTTP, we learned how to expose and invoke Lambda functions over an HTTP endpoint.
We enabled the Function URL without any authentication on the URL endpoint, which makes it possible for anyone with Lambda's URL to invoke the Lambda Function.
When using this in real-world applications, we want to secure the Function URL endpoints and make it possible only for authenticated users or applications to invoke the Lambda Function.
Enable AWS_IAM Authentication Type
When enabling Function URLS, it gives the option to select the Auth type. To restrict the URL to only authenticated users, choose the AWS_IAM Auth type option.
The AWS_IAM option ensures that only authenticated IAM users and roles can make requests to the function URL.
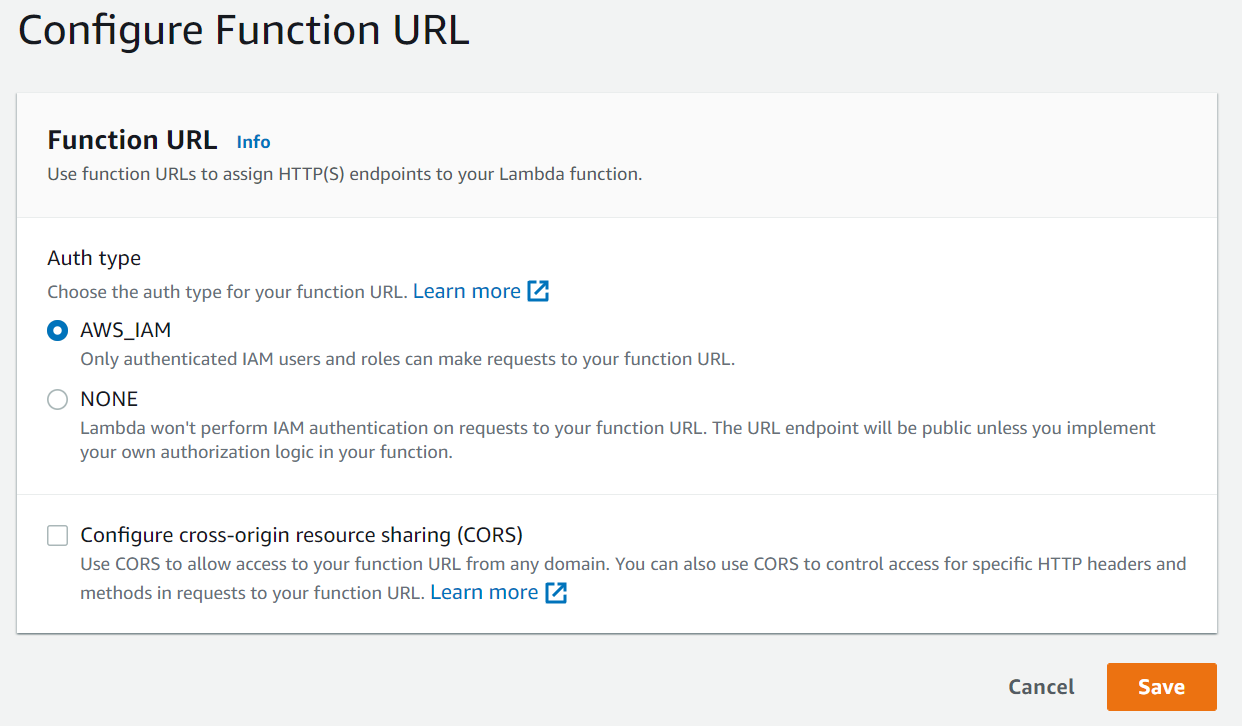
Once enabled, if you try to invoke the Function URL without passing any security headers, it throws a 403 Forbidden request
.
Each HTTP request to an AWS_IAM
enabled Function URL must be signed using AWS Signature Version 4 (SigV4).
Creating an IAM User
The principal requesting the Function URL must either have lambda:InvokeFunctionUrl
permission in their identity-based policy or have permissions granted in the functions resource-based policy.
For identity-based-policy, you can add the InvokeFunction
and InvokeFunctionUrl
permission as shown below.
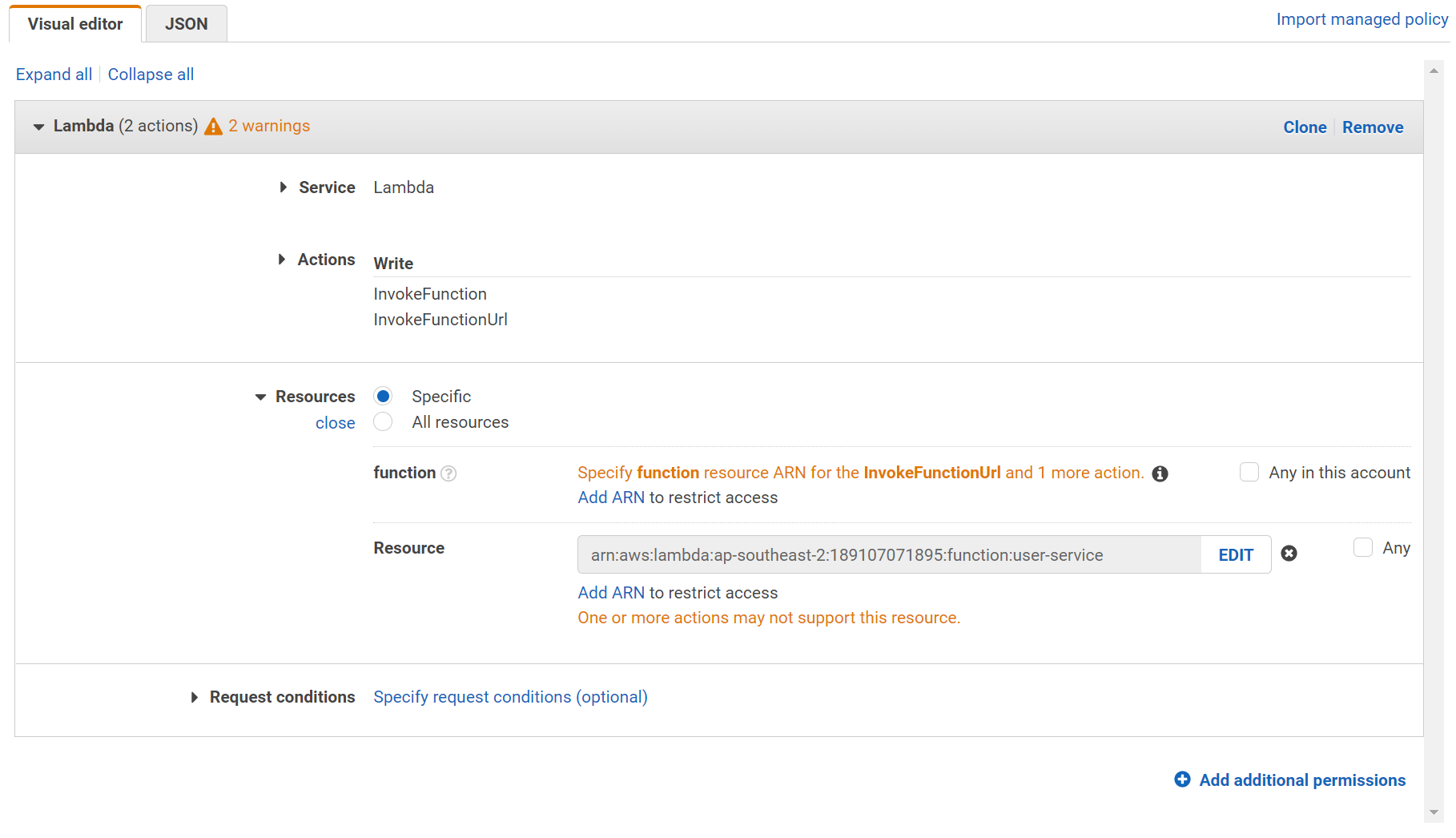
Create an access key for the User with the appropriate permissions to invoke the Function URL. The Access key ID and the Secret are required to sign requests to the Function URL.
Using IAM Credentials From POSTMAN
Postman is an API platform and building APIs. Postman supports authorizing requests using AWS Signature and can be configured to use the AccessKey and Secret for the User from the previous step.
Create a new request in Postman, and under the Authorization tab, choose the Type as AWS Signature
.
It prompts you to enter the AccessKey
and the SecretKey
. It also requires sending the AWS Region
and Service Name
.
In this case, my lambda function is deployed to Sydney (ap-southeast-2) region, so specify AWS Region
as 'ap-southeast-2' and Service Name
as 'lambda'.
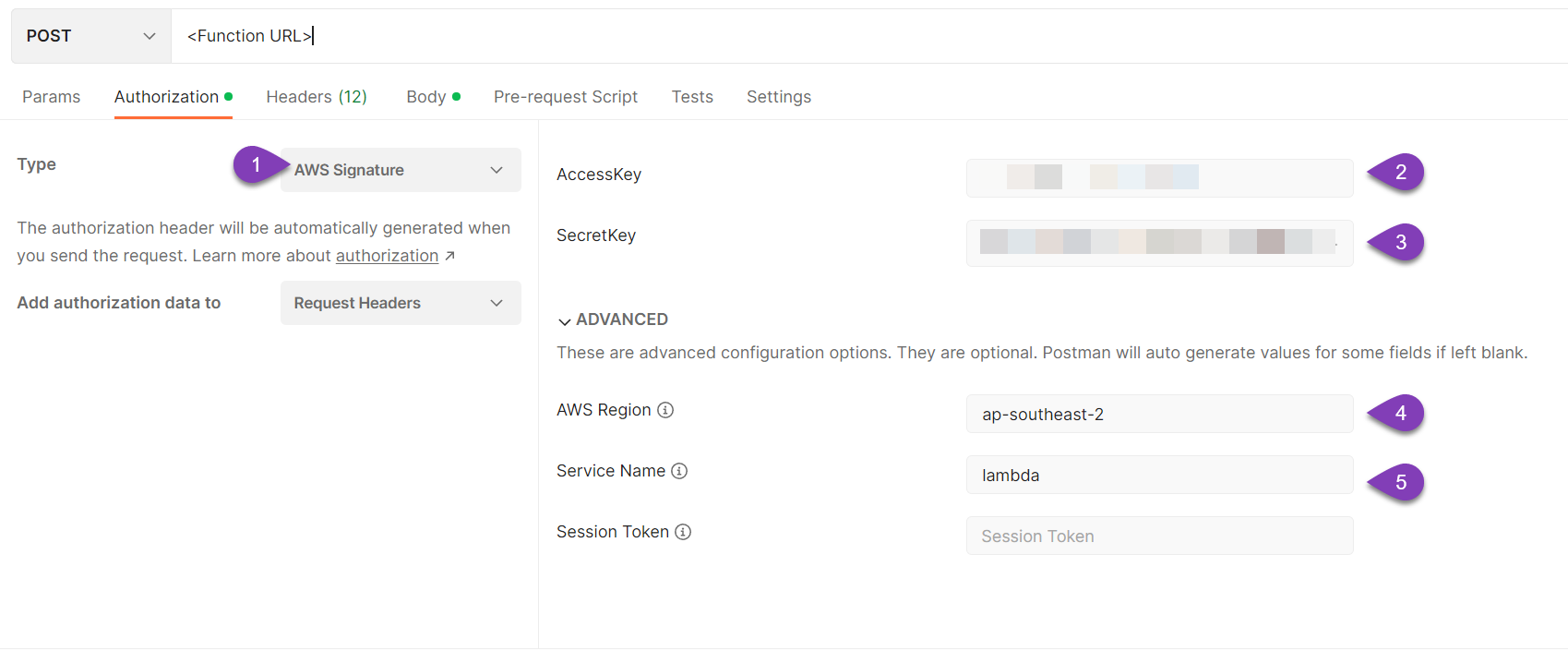
With the AWS Signature setup, you can make a successful request to the AWS Lambda Function URL. Depending on the HTTP Method
specified on the Postman request, it will perform the appropriate action on the Lambda Function.
Calling Function URLs FROM .NET APPs
When building new features, you will have to invoke our Function URL API from other .NET applications.
In .NET, calling other API endpoints is usually done using the HttpClient
class.
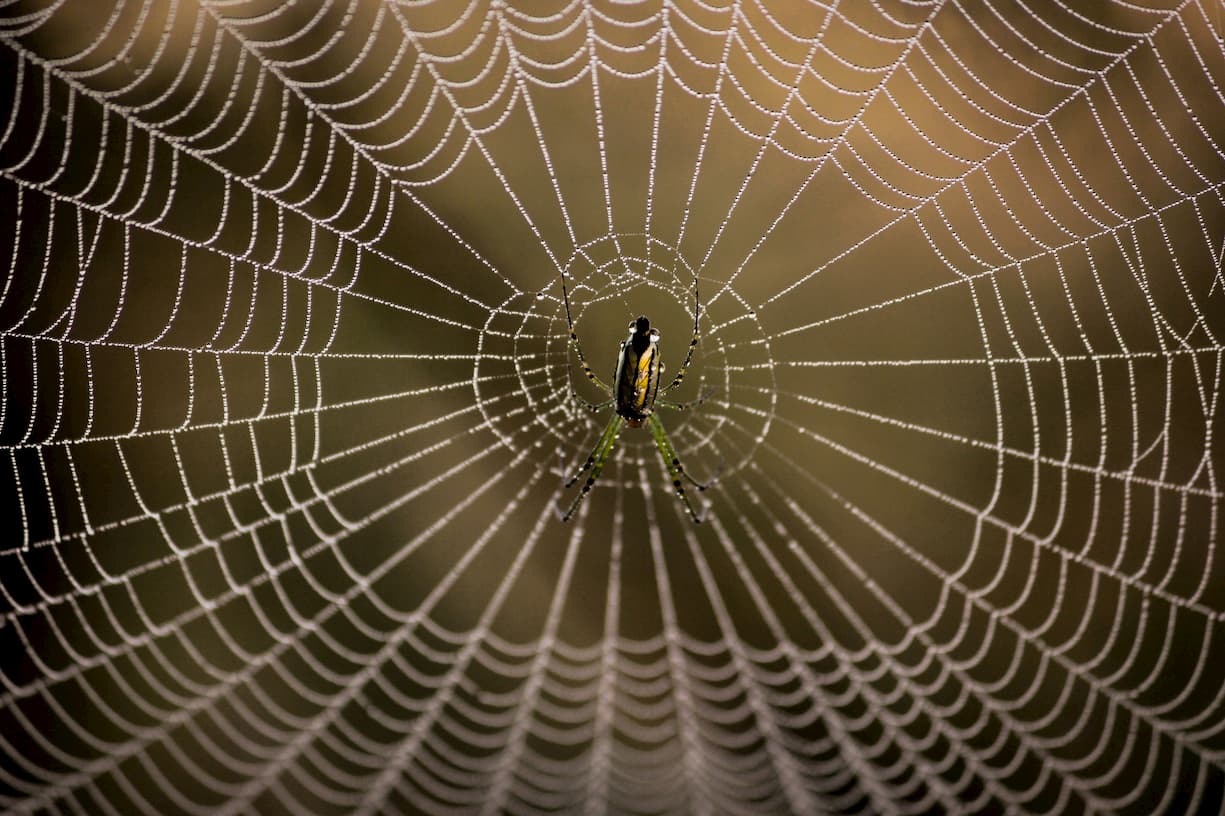
The AwsSignatureVersion4 NuGet package extends the HttpClient
class to add support for AWS Signature 4.
Using AccessKey and Secret, create a new ImmutableCredentials
instance to pass to the different extension methods on the HttpClient
class. You also need to provide the RegionName
and ServiceName
as we did in the Postman example.
Below is the .NET sample code to make an authenticated call to a Lambda Function URL endpoint.
async Task Main()
{
var httpClient = new HttpClient();
var credentials = new ImmutableCredentials(
"ACCESS KEY ID", "SECRET", null);
var result = await httpClient.GetAsync($"https://lrd7ews3klz23s3brksliz7nii0iwsng.lambda-url.ap-southeast-2.on.aws?userId={Guid.NewGuid()}", "ap-southeast-2", "lambda", credentials);
var resultString = await result.Content.ReadAsStringAsync();
result.StatusCode.Dump();
resultString.Dump();
}
Using Environment Credentials
When the .NET applications are already running in an environment where the AWS Credentials are configured, you can use those credentials to invoke the Function URL.
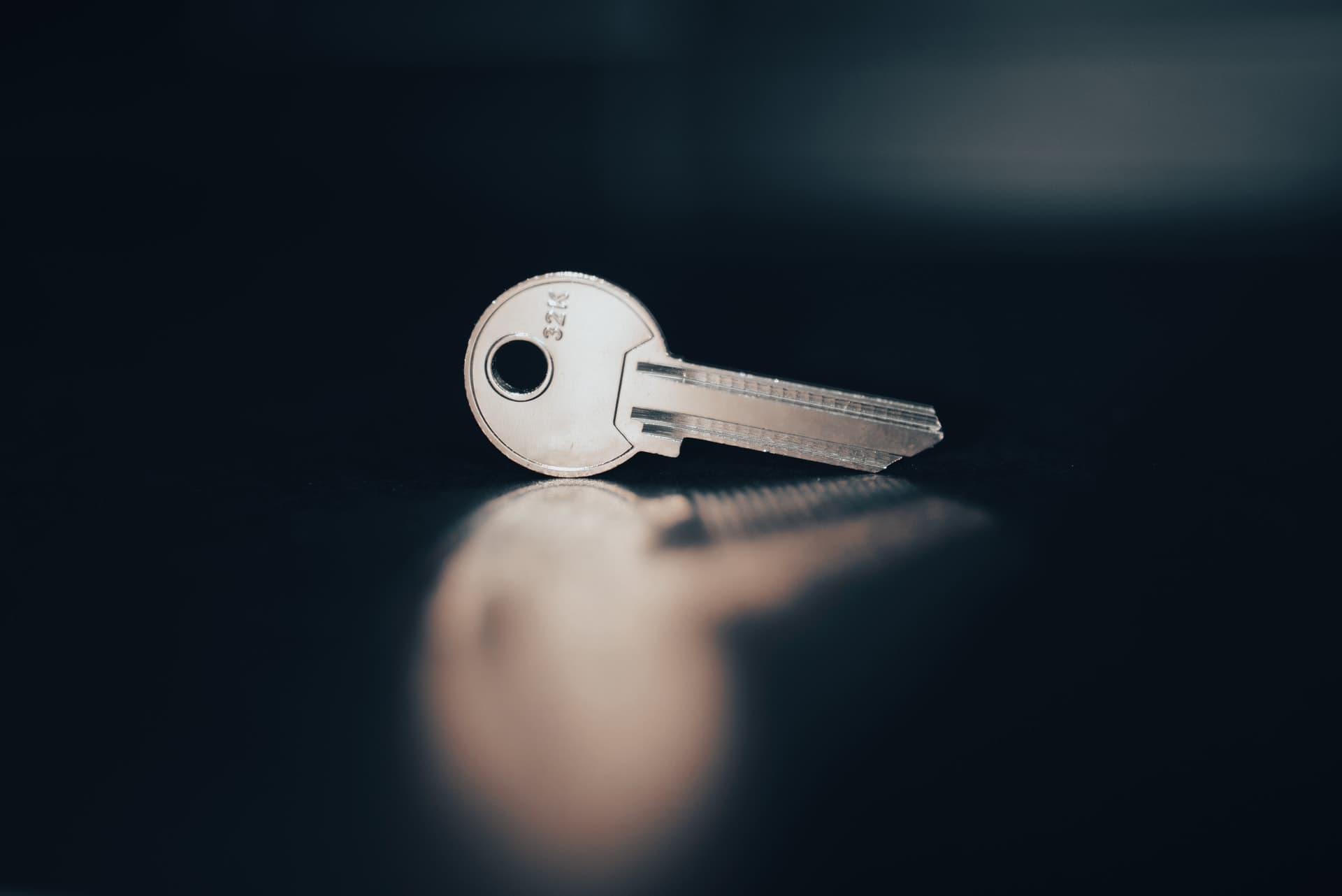
The request will succeed if the configured credentials in the environment have access to invoke the Lambda function URL.
...
var credentials = FallbackCredentialsFactory.GetCredentials();
...
Instead of explicitly creating an ImmutableCredentials
instance from the secret key and access key id, use the FallbackCredentialsFactory
to get the credentials configured at the machine level.
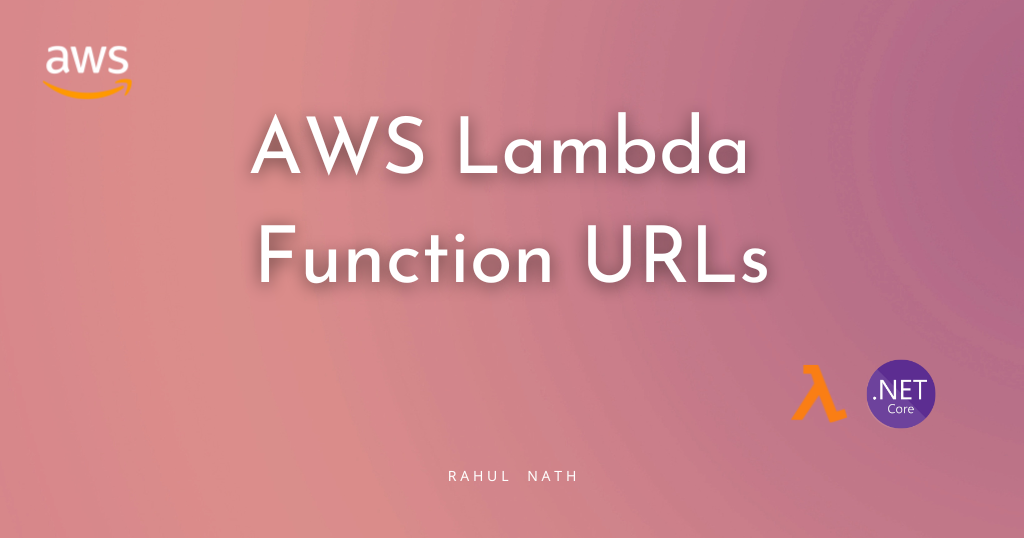
I hope this helps you authenticate your Lambda Function URLs and ensure only authenticated requests are processed on the URL.
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.