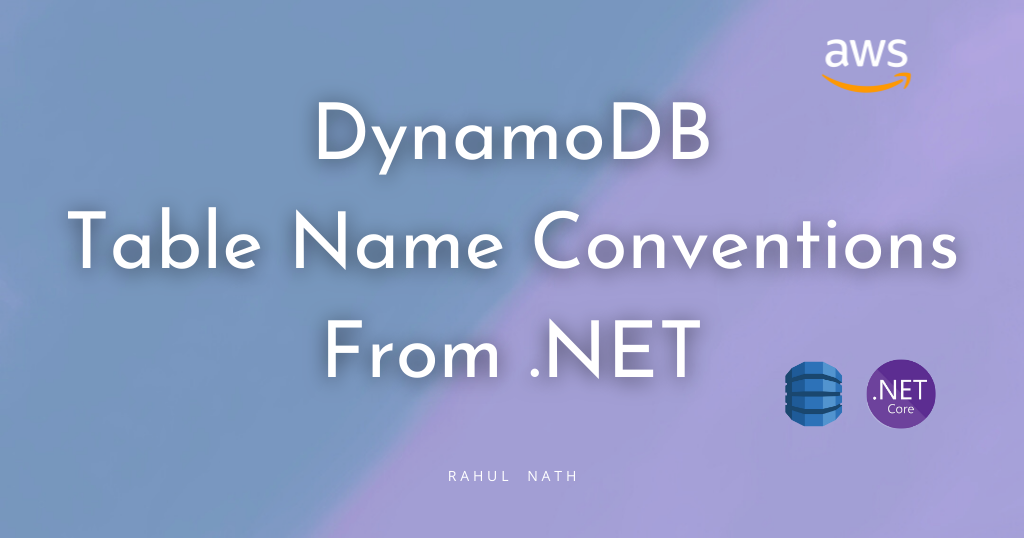
.NET DynamoDB SDK: Understanding Table Name Conventions and DynamoDBTable Attribute
The DynamoDB .NET SDK by default uses conventions to determine the Table name. However, it supports different options to configure and customize the name. Learn how to achieve this in this post.
Table of Contents
The .NET DynamoDB SDK by default uses conventions to resolve Table Names.
The name of the class used in the DynamoDB SDK operations is used as the Table Name by default. For example, if the class is WeatherForecast
, the DynamoDB SDK by default looks for a table with the name 'WeatherForecast'.
However, there are ways you can customize this name and override it based on your application and the environment it is running.
This article is sponsored by AWS and is part of my AWS Series.
In this blog post, let's explore
- Default Table Name Convention Used By DynamoDB .NET
- Configuring DynamoDB Table Name Conventions with .NET
- DynamoDBTableAttribute
If you are new to DynamoDB, I highly recommend checking out my Getting Started with AWS DynamoDB For the .NET Developer blog post below, where I also show you how to set up the table used in this blog post.
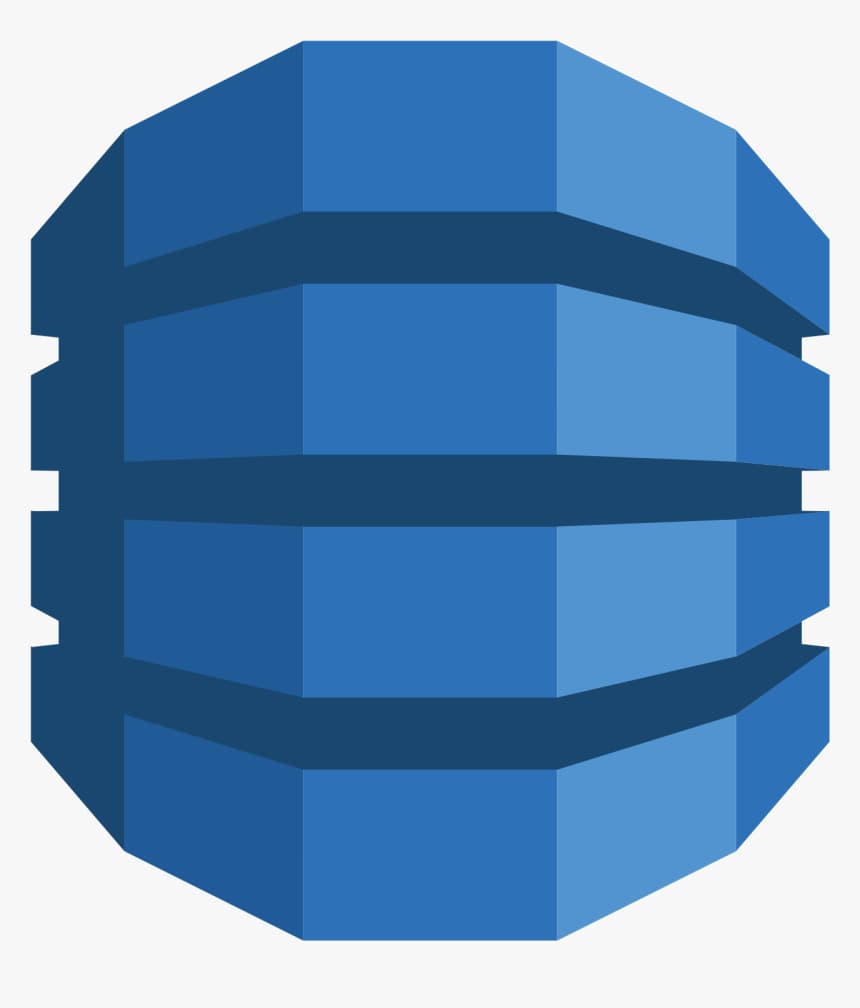
Default Table Name Convention Used By DynamoDB .NET
When using any of the methods on the DynamoDBContext
object on the .NET DynamoDB SDK, you need to pass in a .NET type, as shown below.
return await _dynamoDbContext.LoadAsync<WeatherForecast>(cityName, date);
await _dynamoDbContext
.QueryAsync<WeatherForecast>(cityName).GetRemainingAsync();
await _dynamoDbContext
.QueryAsync<WeatherForecast>(
cityName,
QueryOperator.GreaterThan, new object[] { startDate })
.GetRemainingAsync();
await _dynamoDbContext.SaveAsync<WeatherForecast>(data);
By default, the SDK uses the nameof(WeatherForecast)
to automatically determine the DynamoDB Table Name.
Configuring DynamoDB Table Name Conventions with .NET
Based on your team, application, and AWS account setup you might at times need to override the table names.
One common reason I have come across is when you have multiple application environments (dev, test), etc deployed to the same AWS account. You cannot have multiple tables with the same name in the same AWS account.
In these scenarios, it's great to be able to override/customize the DynamoDB table names based on the application environment/configuration.
For e.g, We can customize our Table names with the environment name they are running on ('dev_WeatherForecast', 'test_WeatherForecast').
You can configure this at the application/global level and also at the individual operation level of the DynamoDBContext
instance.
Application/Global Level
To configure across all the operations on the DynamoDBContext
for an application, you can specify a DynamoDBContextConfig
when creating the DynamoDBContext
.
You can configure this when setting up the DynamoDBContext
in the application DI container.
We can use the TableNamePrefix
property on the configuration, to append the configured text to all the table names.
The below code configures it to 'test_' when the application is running in the test environment.
var dynamoDbClient = new AmazonDynamoDBClient(
FallbackCredentialsFactory.GetCredentials(), RegionEndpoint.APSoutheast2);
var dynamoDBContext = new DynamoDBContext(
dynamoDbClient, new DynamoDBContextConfig() {TableNamePrefix = "test_"});
builder.Services.AddSingleton<IAmazonDynamoDB>(dynamoDbClient);
builder.Services.AddSingleton<IDynamoDBContext>(dynamoDBContext);
The prefix value by itself can come from the application configuration file.
Individual Operation Level
The Table Name conventions can be overridden at individual operation levels as well when using the DynamoDBContext.
The below code specifies the TableNamePrefix
and also the OverrideTableName
properties on the DynamoDBOperationConfig
object.
return await _dynamoDbContext
.QueryAsync<WeatherForecastTable>(
cityName,
new DynamoDBOperationConfig()
{
TableNamePrefix = "test_",
OverrideTableName = "WeatherForecastTable"
})
.GetRemainingAsync();
This overrides the convention-based table name from the type and uses the value specified in the OverrideTableName
property and also prefixes it with the value in the TableNamePrefix
.
If there is a prefix specified at the global level it gets overridden in this case by the one at the operation level. However, you cannot override it to be null or empty at the operation level, if you already specified one at the global level.
DynamoDBTableAttribute
In cases where you want to use a different name for the table outside of the default convention (based on the type name), across all operations on that type, you can use the DynamoDBTableAttribute
.
The DynamoDBTable attribute marks a class and specifies the name to be used by default instead of the one via default convention.
[DynamoDBTable("WeatherForecastTable")]
public class WeatherForecastTable
{
public string CityName { get; set; }
public DateTime Date { get; set; }
...
}
Any time you use this type with the DynamoDBContext
, it uses the name from the DynamoDBTable
attribute if specified on the type.
However, note that any operation level overrides will still take precedence if there is one specified.
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.