
Setting Up Cypress + React App + JSON Server + TypeScript
Table of Contents
For the past couple of weeks, I have been playing around with Cypress and been enjoying the experience. Cypress is a next-generation front end testing tool built for the modern web. It is the next generation Selenium and enables to write tests faster, easier, and reliable.

In this post, let's look at how we can set up Cypress for a React application that runs over a FAKE JSON Server all using TypeScript. By using a Fake Server for the tests, we can guarantee the application state and the data to expect.
Create React App
Setting up a Create React App with TypeScript is straightforward and supported out of the box. All you need to specify is the typescript template when you create a new application (as shown below). The documentation also has steps on how to add Typescript to an existing project.
npx create-react-app my-app --template typescript
Setting Up JSON Server
In the previous post we looked at how to set up a Fake REST API using JSON Server. Let's move JSON Server and the mock data to TypeScript. It forces us to update the mock data any time the models are updated. I have JSON Server under the mockApi folder.
npm install json-server @types/json-server typescript
Add a tsconfig.json for the TypeScript compiler and update the server.js file to server.ts.
{
"compilerOptions": {
"module": "commonjs",
"target": "es6",
"moduleResolution": "node",
"esModuleInterop": true,
"lib": ["es6"],
"forceConsistentCasingInFileNames": true
},
"include": ["**.ts"]
}
// server.ts
import jsonServer from 'json-server';
import data from './mockData';
const server = jsonServer.create();
const router = jsonServer.router(data);
...
server.use(router);
server.listen(5000, () => {
console.log('JSON Server is running');
});
For the mock data, use the API Model DTO type definitions if you have a swagger definition for the API's, use NSwag to generate TypeScript definitions. Generating the definitions can be scripted or be done using the NSwag Studio.
const quotes: QuoteDto[] = [
{
id: "1",
statusCode: QuoteStatusCode.Draft,
lastModifiedAt: new Date("2-Mar-2020"),
customer: {...},
mobilePhone: null,
accessories: [],
},
];
'npx ts-node server.ts' Start Mock API
Depending on how you have the React app calling the API, you can set it up to use the Mock API Server that we are running. If the Web App and API are served from the same host and port usually, you can proxy the requests to JSON Server by setting the proxy field in package.json.
Setting Up Cypress
The Cypress docs are well explained and have a step by step walkthrough to set up Cypress tests. I have Cypress installed under the web application folder.
- Set up Cypress
- Enable Typescript support for Cypress. Add tsconfig.json under Cypress folder.
- Install and Set up Cypress Testing Library. Types are available at DefinitelyTyped
npm install --save-dev cypress @testing-library/cypress @types/testing-library__cypress
Cypress comes with default test examples. If the example tests are not showing up for you, try running 'cypress open' (or run), which should generate them. You can ignore the tests from running in the cypress.json file.
{
"ignoreTestFiles": "**/examples/*.js",
"baseUrl": "http://localhost:3000"
}
Below is the folder structure that I have - mockApi (JSON Server), cypress, and ui (create-react-app).
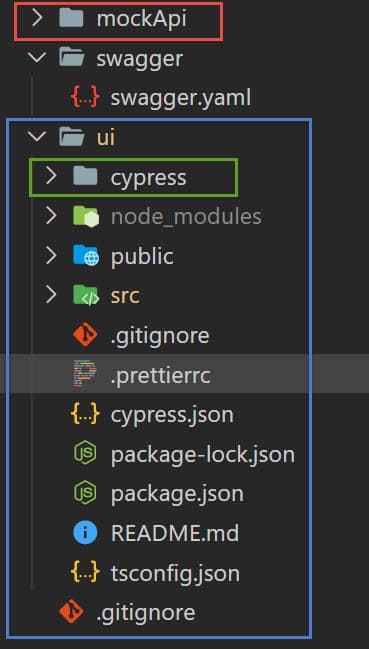
Start writing Cypress tests now!
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.