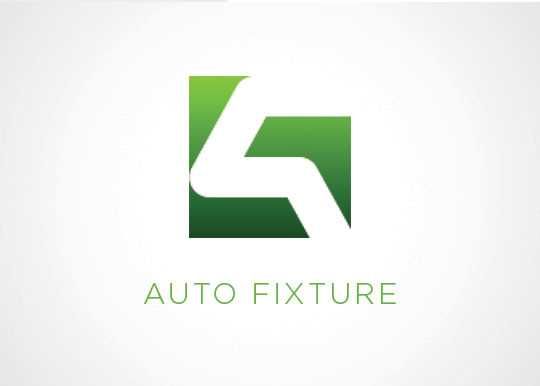
Tip of the Week: AutoFixture - Make Your Unit Tests Robust
AutoFixture is an open source library for .NET designed to minimize the 'Arrange' phase of your unit tests in order to maximize maintainability
Table of Contents
AutoFixture is an open source library for .NET designed to minimize the 'Arrange' phase of your unit tests in order to maximize maintainability. Its primary goal is to allow developers to focus on what is being tested rather than how to setup the test scenario, by making it easier to create object graphs containing test data.
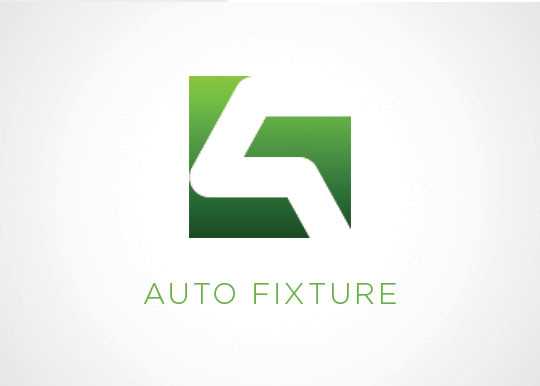
If you are on .NET platform and write tests (there is no reason you wouldn't) you should check out AutoFixture. AutoFixture makes test data setup easy. It is a generalization of the Test Data Builder pattern and helps make your tests more robust and maintainable. Below is a sample (as taken from the GitHub page) shows how minimal setup is required for testing. Check out the post, Refactoring Test Code: Removing Constructor Dependency to see in detail how AutoFixture can be used to make the tests more stable against changes.
[Theory, AutoData]
public void IntroductoryTest(
int expectedNumber, MyClass sut)
{
int result = sut.Echo(expectedNumber);
Assert.Equal(expectedNumber, result);
}
If you are new to AutoFixture, I highly recommend checking out the Cheat Sheet to get started. Check out my post on Populating Data for Tests for some common patterns of using AutoFixture and how it can reduce setup code. Understanding the Internal Architecture of AutoFixture helps if you want to extend it for customization. AutoFixture integrates well with the different testing frameworks and support libraries that are popular. I mostly use it with xUnit and Moq.
Hope this helps with your testing!
I am happy to have contributed (minor) to such a great library.
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.