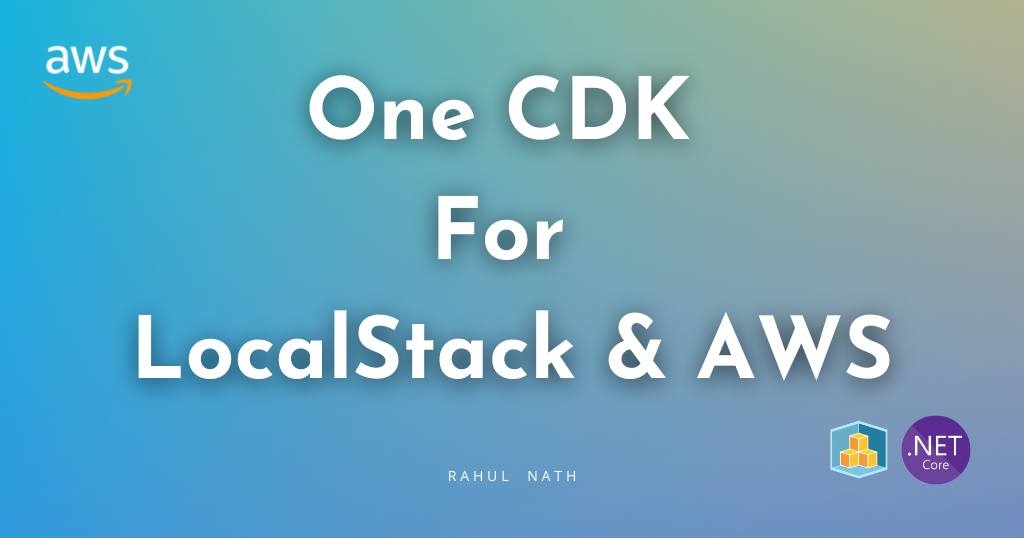
Setting Up AWS CDK with .NET for Seamless LocalStack and AWS Deployments
Let's learn how to use CDK to deploy and manage resources in both LocalStack and AWS accounts. We will use the same CDK code base to deploy to both.
Table of Contents
Developing and testing AWS applications locally can be challenging without the right tools.
AWS CDK makes deploying and managing resources effortless.
And when combined with LocalStack, it allows for a smooth local development experience.
In this post, we'll explore
- Setup CDK
- Deploy CDK To LocalStack
- Deploy CDK to AWS Cloud
Thanks to AWS for sponsoring this post in my .NET on AWS Series
Setting up CDK
For this post, I will deploy a simple ASP NET Web API application that writes data for DynamoDB and publishes it for SQS.
I also have a Lambda Function that listens for messages on the SQS and is triggered whenever a new message comes into the queue.
If you are new to CDK, check out my blog post below to learn some of its core concepts.
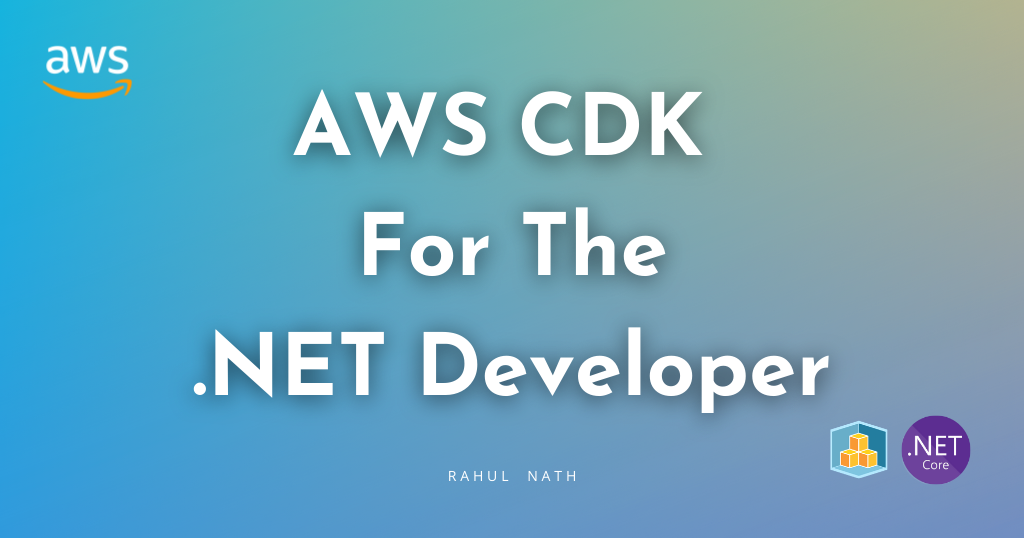
The CDK code below sets up the Web API on a Lambda Function, sets up the DynamoDB table and SQS queue, and gives appropriate permissions for the API lambda function.
It also sets up a Lambda Function to listen to SQS messages and the required triggers for invoking the function on a new SQS message.
var weatherForecastTable = new Table(this, "WeatherForecastData",
new TableProps{...});
var weatherDataQueue = new Queue(this, "WeatherDataQueue", new QueueProps{...});
var weatherLambdaFunction = new Function(this, "WeatherDataProcessor",
new FunctionProps{});
weatherForecastTable.GrantReadWriteData(weatherLambdaFunction);
weatherLambdaFunction.AddEventSource(new SqsEventSource(weatherDataQueue));
var environmentVariables = new Dictionary<string, string>();
var environment = this.Node.TryGetContext("ASPNETCORE_ENVIRONMENT")?.ToString();
if (!string.IsNullOrEmpty(environment))
{
Console.WriteLine("Setting ASPNETCORE_ENVIRONMENT variable");
environmentVariables.Add("ASPNETCORE_ENVIRONMENT", environment);
}
var apiLambdaFunction = new Function(this, "WeatherForecastApi", new FunctionProps
{
...
Environment = environmentVariables
});
var functionUrl = apiLambdaFunction.AddFunctionUrl(new FunctionUrlOptions
{
AuthType = FunctionUrlAuthType.NONE
});
new CfnOutput(this, "API FunctionUrl", new CfnOutputProps
{
Value = functionUrl.Url
});
weatherForecastTable.GrantReadWriteData(apiLambdaFunction);
weatherDataQueue.GrantSendMessages(apiLambdaFunction);
Above is the high-level code (only placeholders) to set up our application. The full source code for the CDK Stack can be found here.
Deploy CDK To LocalStack
LocalStack is a fully functional local AWS cloud stack that lets you emulate AWS services directly on your machine.
If you are new to LocalStack check out the article below to get up and running.
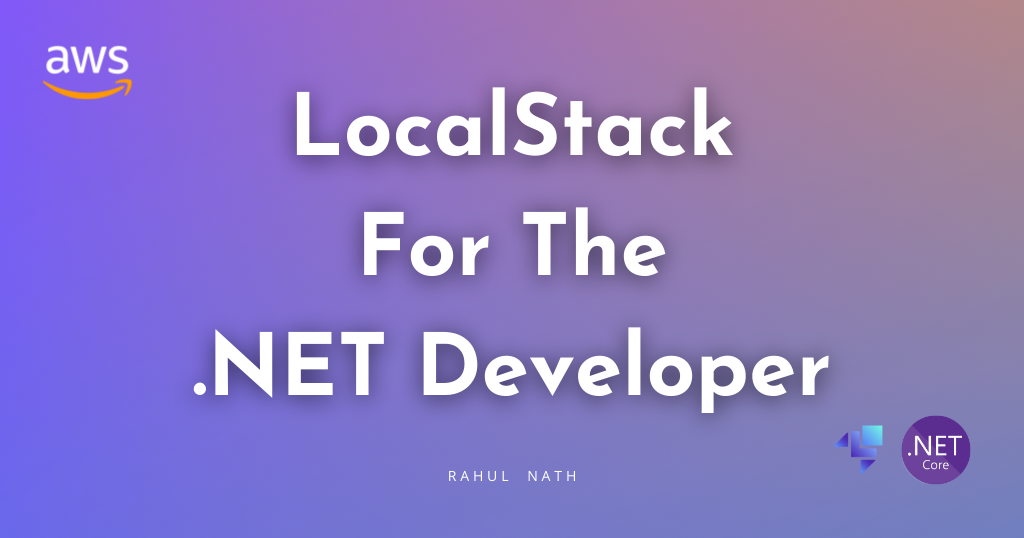
The aws-cdk-local provides a wrapper script cdklocal
that you can use to deploy CDK resources to localstack.
This can be installed as a npm package.
$ npm install -g aws-cdk-local aws-cdk
...
$ cdklocal --version
To deploy CDK onto LocalStack, we first need to bootstrap the account using the same method we use to bootstrap AWS accounts.
cdklocal bootstrap
Once bootstrapped, you can deploy a CDK stack using the deploy command
cdklocal deploy
Overriding .NET Configuration to run in LocalStack
In the Getting Started with LocalStack article, I showed different ways to configure .NET app to work with LocalStack.
When running applications within LocalStack that needs to talk to other resources within LocalStack, you need to make sure that the ServiceURL
is updated to use the docker special host address - host.docker.internal
.
Now to make this to work we can add a new appsettings file - appsettings.LocalStack.json
and add in the configuration as below.
"AWS": {
"Region": "ap-southeast-2",
"ServiceURL": "http://host.docker.internal:4566",
"AuthenticationRegion": "ap-southeast-2"
}
appsettings.LocalStack.json file
Now, we need to ensure that when our application runs within the LocalStack environment, it uses this app settings configuration file.
For this, we can set the ASPNETCORE_ENVIRONMENT
(or DOTNET_ENVIRONMENT
) to specify the application environment.
We can use CDK Context to pass in additional parameter when running on local development machine to set the appropriate environment value.
cdk.json
or cdk.context.json
in your project directory) or on the command line.The below CDK code looks for the ASPNETCORE_ENVIRONMENT
in the context, and if one exists, it adds that value to the Environment Variable for the lambda function.
var environmentVariables = new Dictionary<string, string>();
var environment = this.Node.TryGetContext("ASPNETCORE_ENVIRONMENT")?.ToString();
if (!string.IsNullOrEmpty(environment))
{
Console.WriteLine("Setting ASPNETCORE_ENVIRONMENT variable");
environmentVariables.Add("ASPNETCORE_ENVIRONMENT", environment);
}
var apiLambdaFunction = new Function(this, "WeatherForecastApi", new FunctionProps
{
...
Environment = environmentVariables
});
This ensures that when the application runs within the Lambda Function in LocalStack, it will use the appsettins.LocalStack.json
file.
You can pass the parameter in cdklocal
command as below.
cdklocal deploy -c ASPNETCORE_ENVIRONMENT=LocalStack
Deploy CDK to AWS Cloud
Once you have tested your application end to end on your local development machine on LocalStack, you can easily push it to AWS Cloud.
You don't need to set the additional environment variable for this, and the application code and CDK will work as is.
All you need to run
.... is cdk deploy
You will have all the resources and applications set up and running on AWS Cloud.
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.