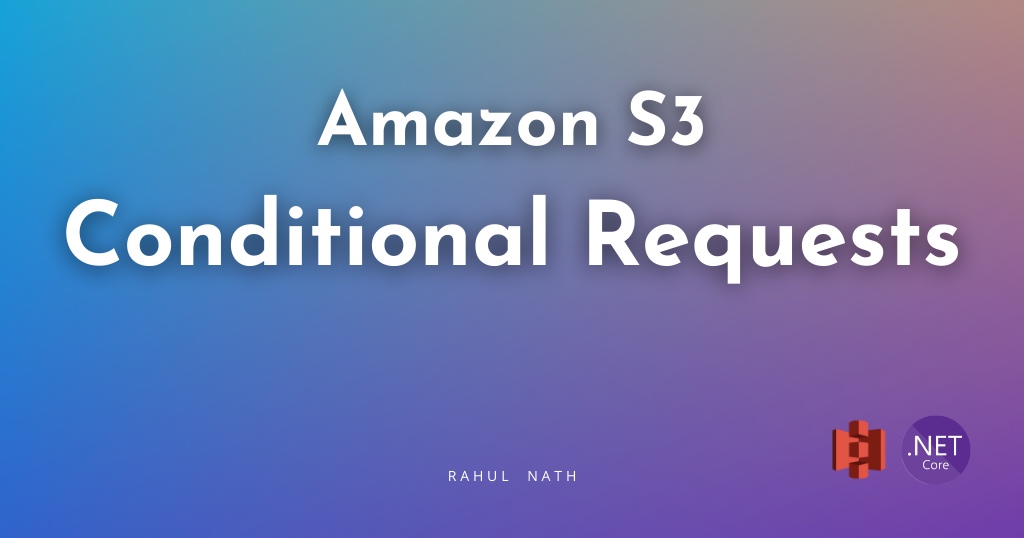
Exploring Amazon S3 Conditional Operations From .NET Application
Amazon S3 now supports conditional requests. You can use conditional requests to add preconditions to your S3 operations. If the precondition is not met it will result in the S3 operation failing. Let’s learn about Conditional Reads and Writes in Amazon S3 and how to use it from .NET
Table of Contents
Amazon S3 now supports conditional requests.
You can use conditional requests to add preconditions to your S3 operations. If the precondition is not met, the S3 operation will fail.
There is no additional charge for conditional requests.
You are only charged existing rates for the applicable requests, including for failed requests.
Conditional reads and writes in Amazon S3 help ensure data consistency and prevent conflicts in concurrent environments.
By specifying conditions you can avoid accidental overwrites, stale reads etc helping maintain data integrity.
Let’s explore both Conditional Reads and Conditional Writes in Amazon S3 and how to use them when building .NET applications.
This article is sponsored by AWS and is part of my .NET on AWS Series.
Amazon S3 Conditional Writes From .NET
Amazon S3 Conditional writes check for the existence of an object during the write operation.
The write operation will fail if an identical key name is found in the bucket.
This allows multiple clients to write to the same bucket without the risk of overwriting existing objects.
The PutObject
and CompleteMultipartUpload
API method supports conditional writes.
When making the API request, specify the If-None-Match
HTTP header with the value '*' (asterisk).
To use this from the .NET AWS S3 SDK, specify the IfNoneMatch
property on the PutObjectRequest
as shown below.
await s3Client.PutObjectAsync(new PutObjectRequest()
{
BucketName = "<bucket-name>",
Key = request.File.FileName,
InputStream = request.File.OpenReadStream(),
IfNoneMatch = "*"
}, cancellationToken);
If a file with the same name exists in the bucket, the PutObjectRequest
will fail with an exception - Amazon.S3.AmazonS3Exception: At least one of the pre-conditions you specified did not hold
If a file does not already exist, it is uploaded successfully.
Amazon S3 Conditional Writes and Bucket Versioning
Amazon S3 versioning is a powerful feature that allows you to preserve, retrieve, and restore every version of every object in your bucket.
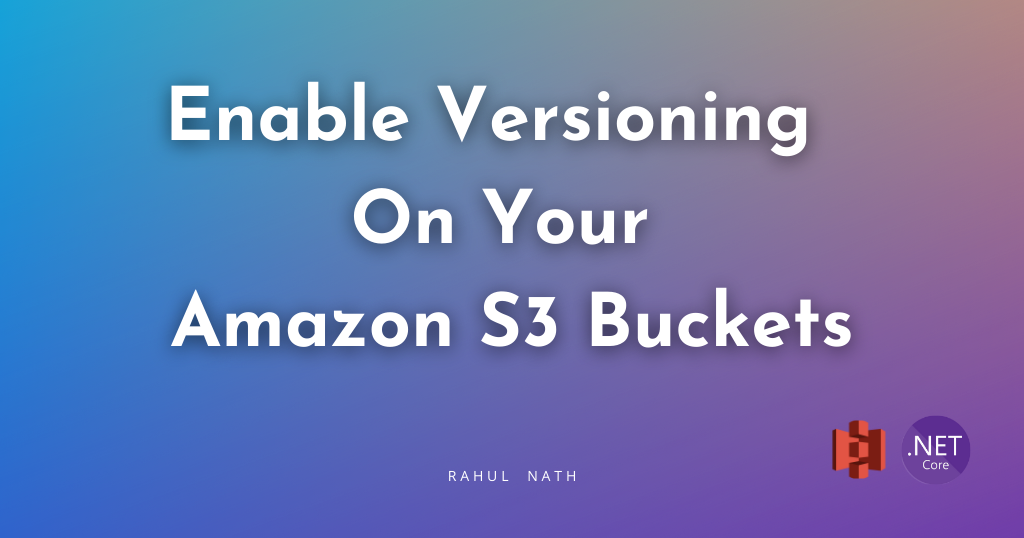
Conditional Requests work with versioning-enabled S3 buckets, too.
A new file is written only if a file with same name does not exists or if the current object version is a delete marker.
Amazon S3 Conditional Reads From .NET
With conditional reads in Amazon S3, you can return an object based on the object's metadata (ETag or Last-Modified date).
Whenever an object is uploaded to Amazon S3, it automatically creates system-controlled metadata.
An object's ETag is a string that represents its specific version. The Last-Modified date is metadata indicating either the object's creation date or its most recent modification, whichever is later.
Amazon S3 API supports conditional reads on GET
, HEAD
or COPY
requests.
Conditional reads allow you to retrieve an object based on its ETag or Last-Modified date.
By specifying an ETag in your request, the object will be returned only if the ETag matches, ensuring that you retrieve or copy a specific version.
Similarly, by providing a Last-Modified date, the object will only be returned if modified after the specified date.
Below are the various HTTP headers supported with conditional reads.
- If-Match: Returns object only if its ETag matches the provided value.
- If-Modified-Since: Returns object only if it has been modified after the specified date.
- If-None-Match: Returns object only if its ETag does not match the provided value.
- If-Unmodified-Since: Returns the object only if it has not been modified after the specified date.
To use this from the .NET AWS S3 SDK, specify the IfNoneMatch
property on the GetObjectRequest
as shown below.
var file = await s3Client.GetObjectAsync(new GetObjectRequest()
{
BucketName = "user-service-large-messages",
Key = fileName,
EtagToMatch = etag
});
The above code returns a file only if the ETag value provided matches that on S3.
Similarly, you can use EtagToNotMatch
, ModifiedSinceDateUtc
or UnmodifiedSinceDateUtc
property to specify read preconditions, depending on your use case.
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.