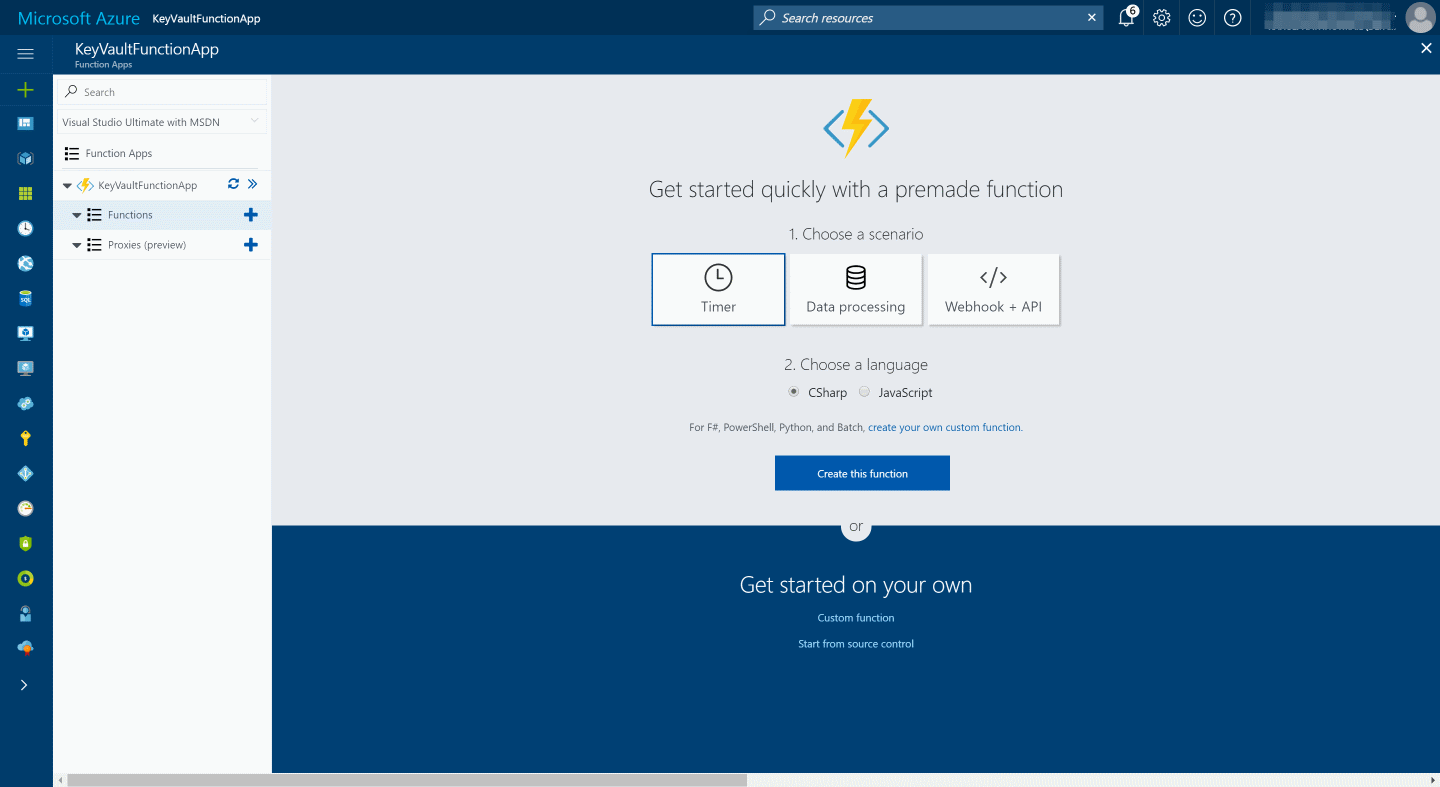
Azure Key Vault From Azure Functions
How to access Key Vault objects from Azure Function.
Table of Contents
Azure Functions is a solution for easily running small pieces of code, or "functions," in the cloud. You can write just the code you need for the problem at hand, without worrying about a whole application or the infrastructure to run it. Functions can make development even more productive, and you can use your development language of choice, such as C#, F#, Node.js, Python or PHP. Pay only for the time your code runs and trust Azure to scale as needed. Azure Functions lets you develop serverless applications on Microsoft Azure.
Even when developing with Azure Functions you want to keep your sensitive data protected. Like for example if the function needs to connect to a database you might want to get the connection string from Azure Key Vault. If you are new to Azure Key Vault check out these posts to get started. In this post, we will explore how we can consume objects in Azure Key Vault from an Azure Function.
Create Azure Function App: Let's first create an Azure Function App from the Azure portal. Under New - Compute - Function App you can create a new Azure Function.
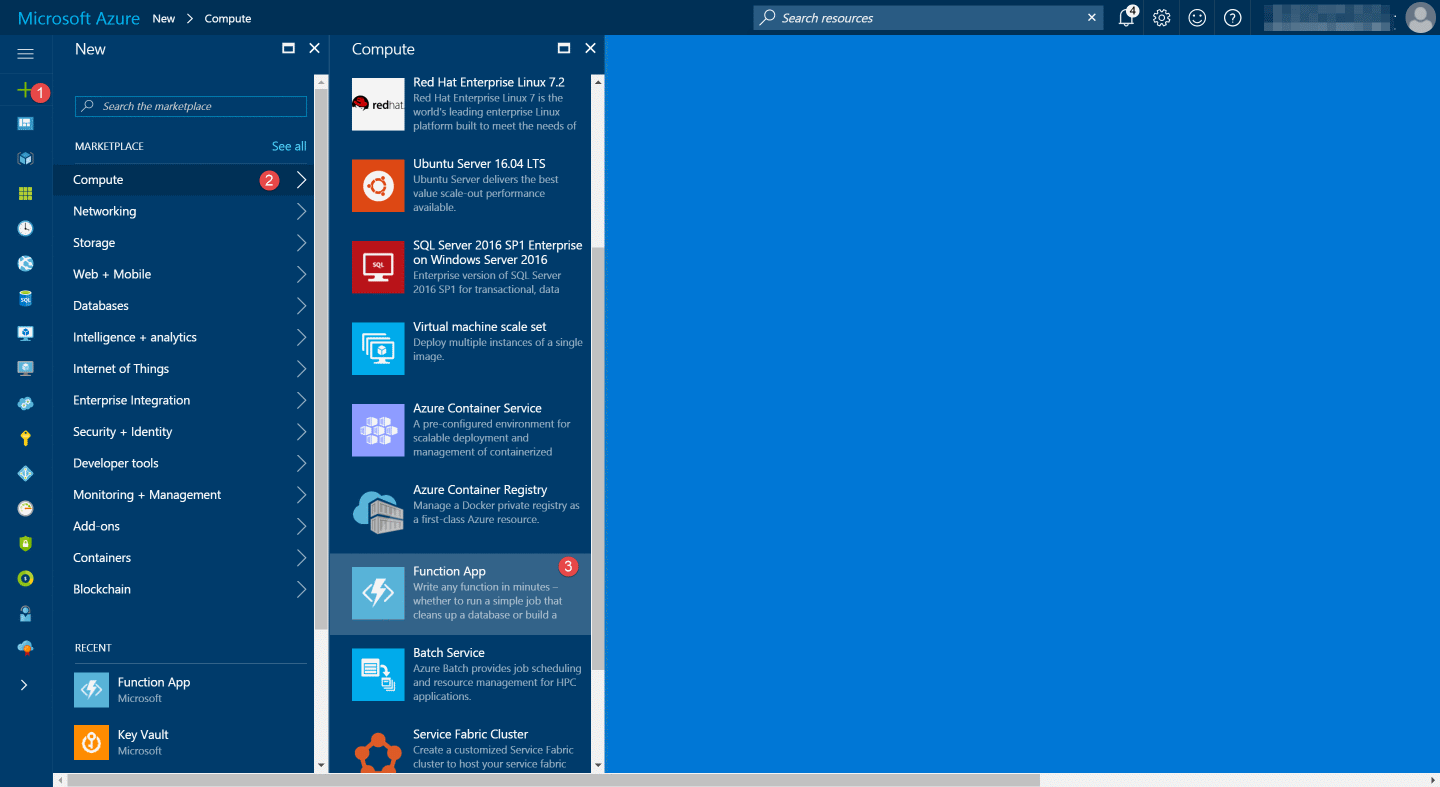
Enter the details of the new function app and press Create. Each function app has an associated storage account. You can choose an existing one or create a new one.
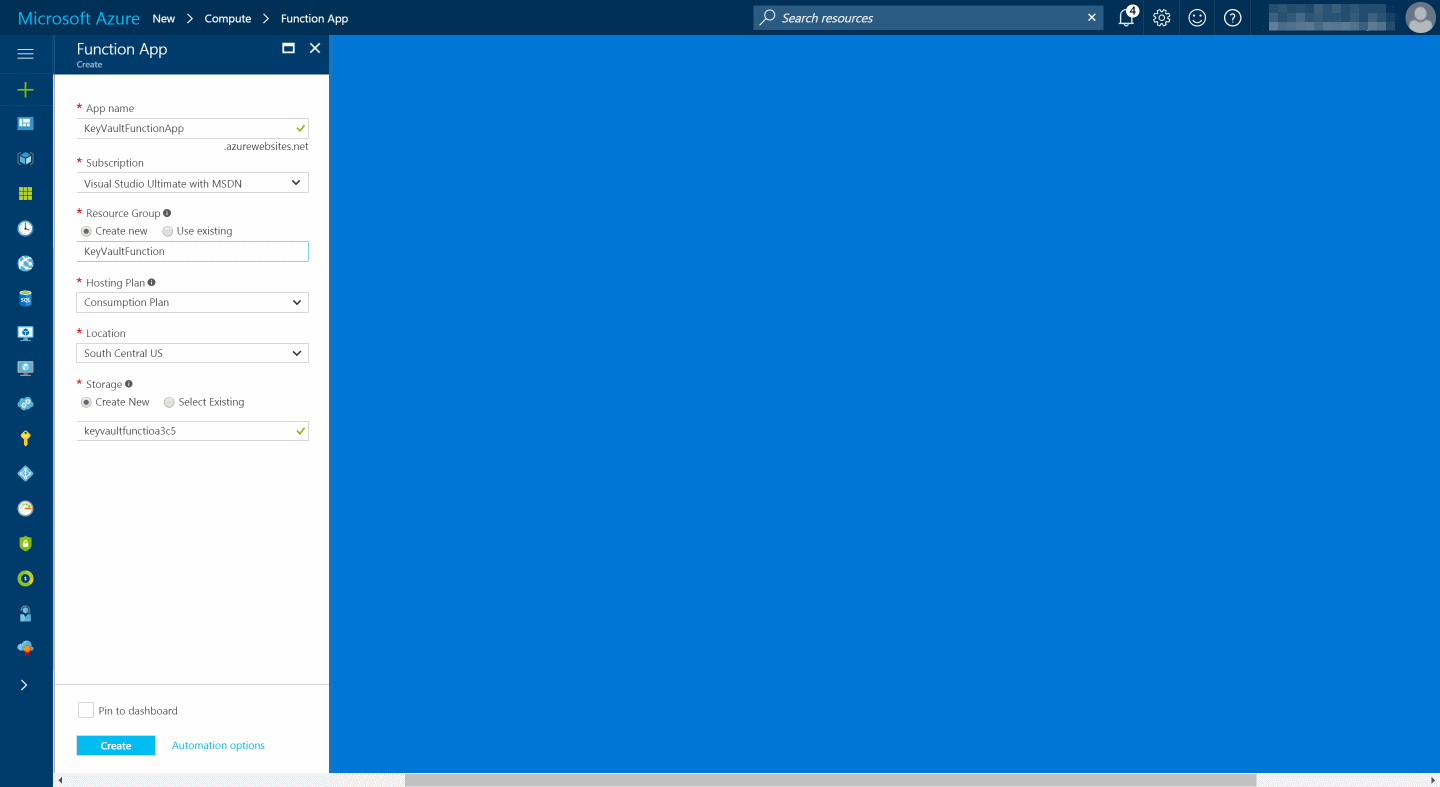
You can view all Azure Functions Apps in the subscription under More services - Function Apps
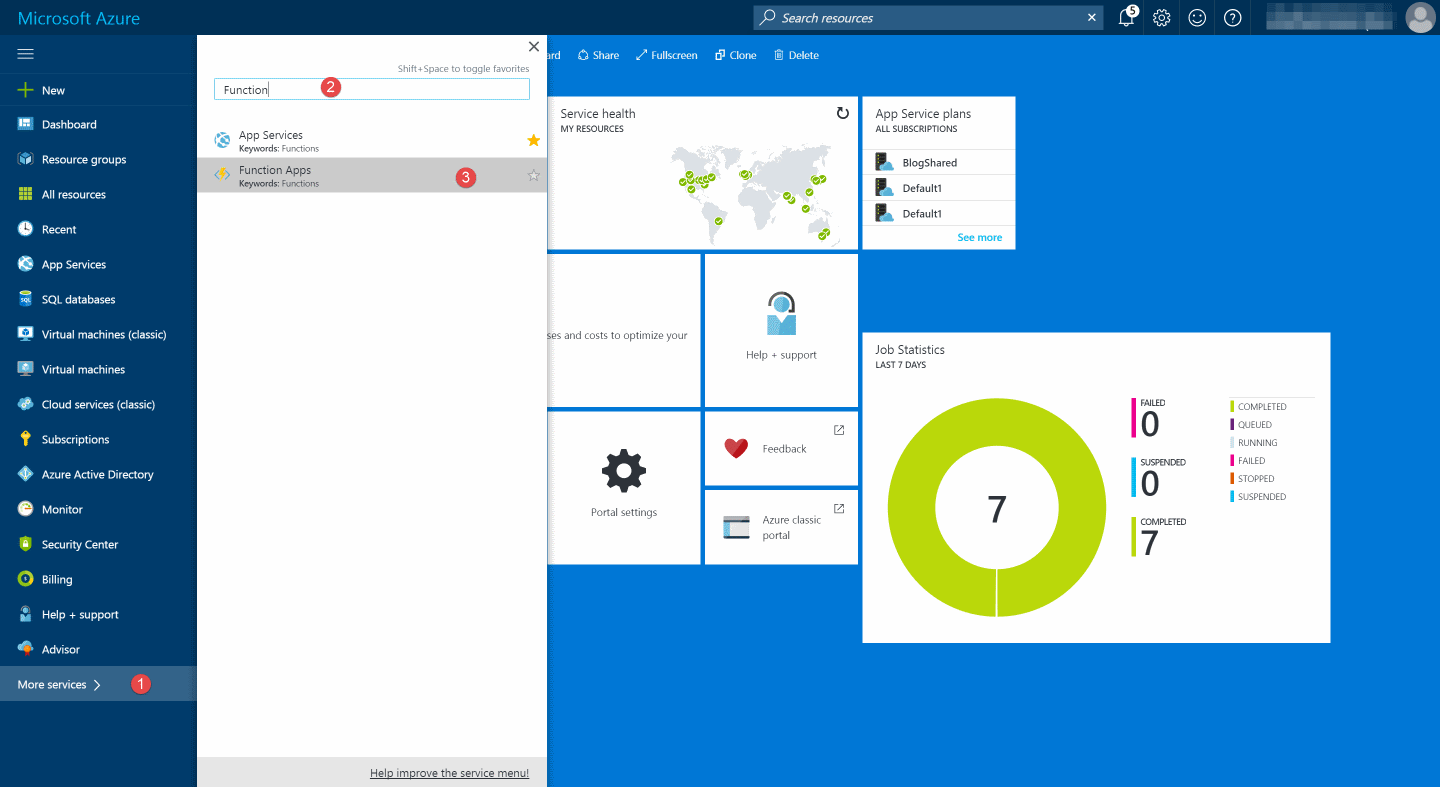
Create Function: To create a function you can create from an existing template or create a custom function. In this example, I will use a timer based function in C#.
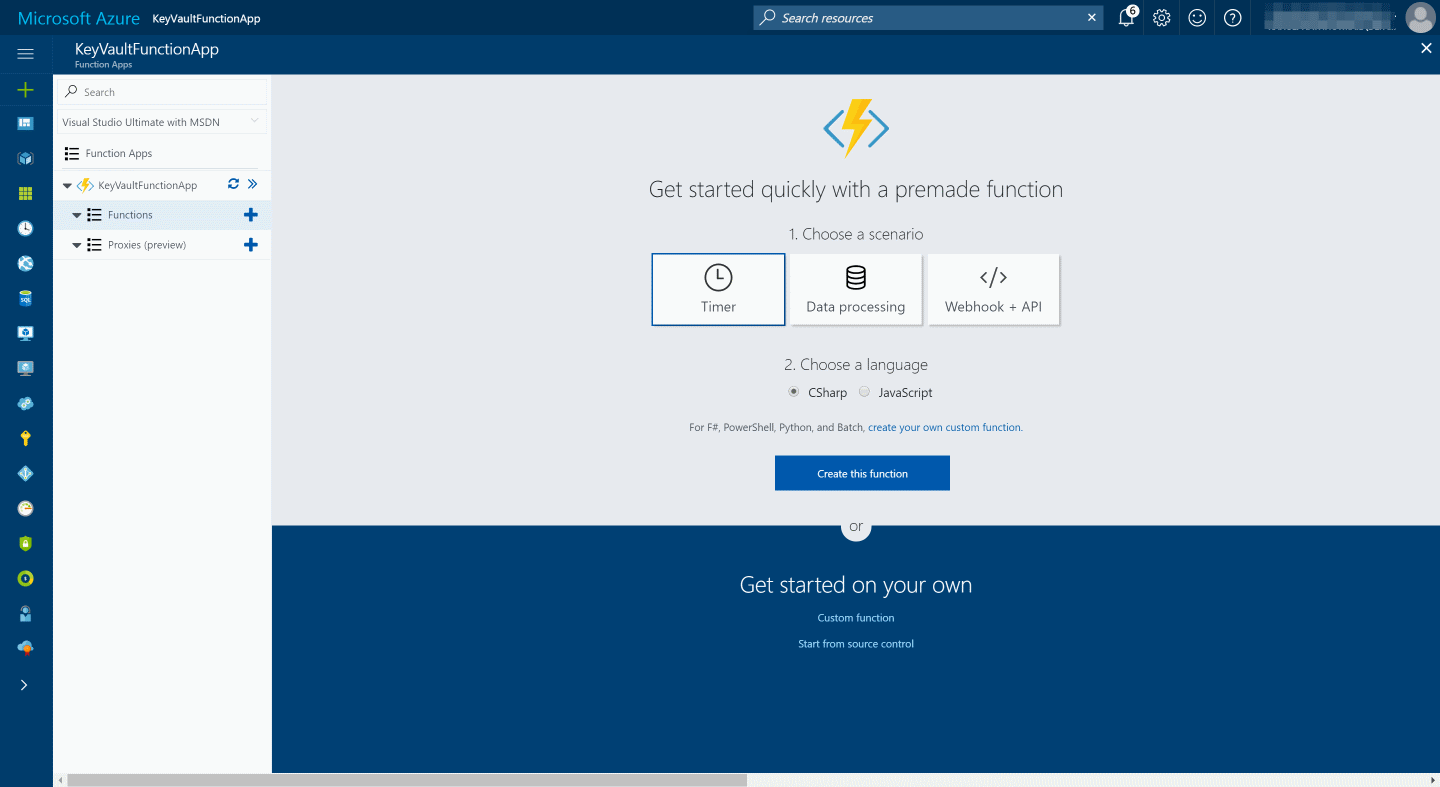
In the run.csx file add in the code for the function. The below code fetches the secret value from the Key Vault and logs it. You need to provide the Azure AD Application Id and secret to authenticate with it. Alternatively you can also use certificate based authentication to authenticate with the Key Vault. Make sure you add in the relevant using statements for the KeyVault client Azure Active Directory Authentication libraries (ADAL).
using System;
using Microsoft.Azure.KeyVault;
using Microsoft.IdentityModel.Clients.ActiveDirectory;
private const string applicationId = "AD Application Id";
private const string applicationSecret = "AD Application Secret";
public async static Task Run(TimerInfo myTimer, TraceWriter log)
{
var keyClient = new KeyVaultClient(async (authority, resource, scope) =>
{
var adCredential = new ClientCredential(applicationId, applicationSecret);
var authenticationContext = new AuthenticationContext(authority, null);
return (await authenticationContext.AcquireTokenAsync(resource, adCredential)).AccessToken;
});
var secretIdentifier = "https://rahulkeyvault.vault.azure.net/secrets/mySecretName";
var secret = await keyClient.GetSecretAsync(secretIdentifier);
log.Info($"C# Timer trigger function executed at: {secret}");
}
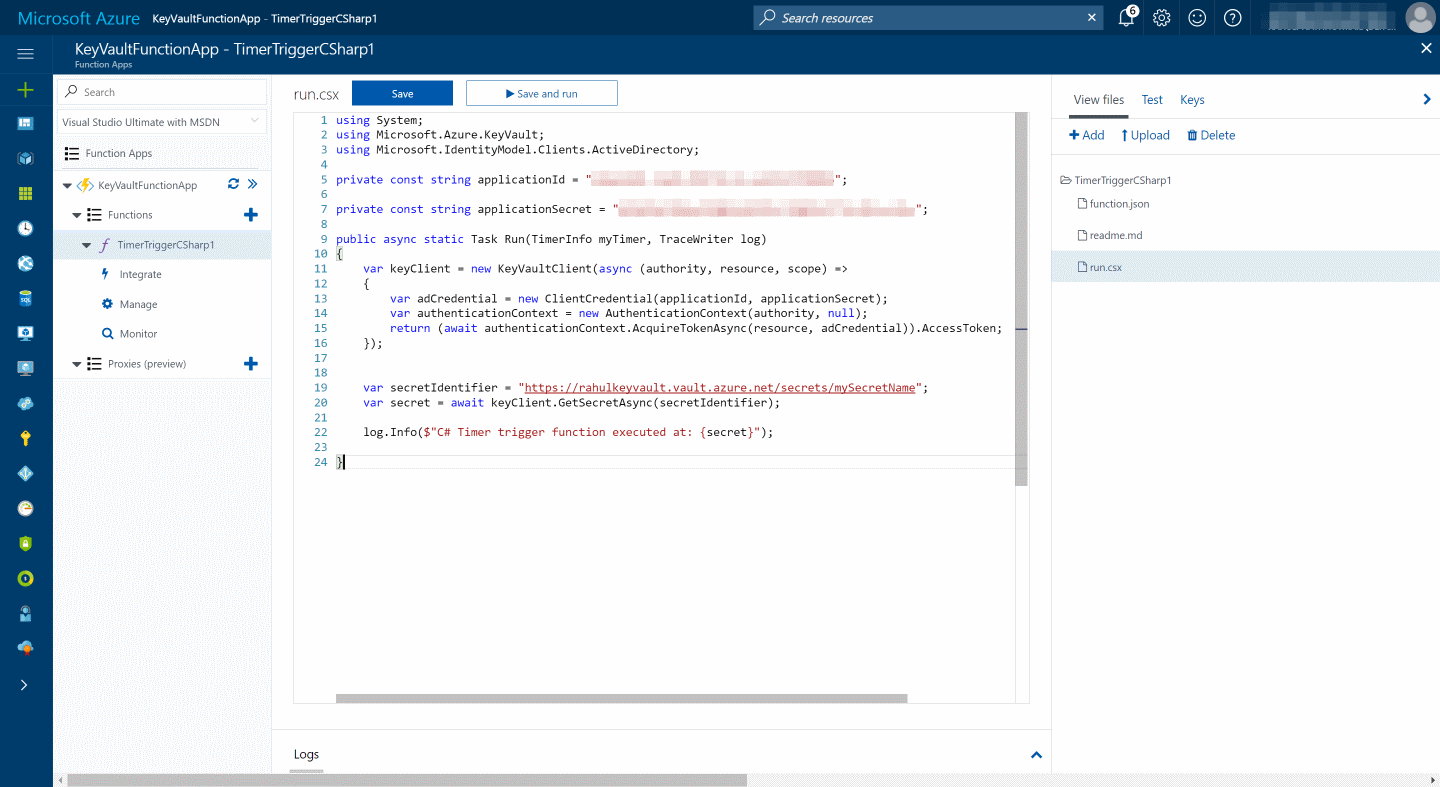
Since the KeyVaultClient and the ADAL libraries are NuGet packages, we need to specify these as dependencies for the Azure Function. To use NuGet packages, create a project.json file in the functions folder. Add in both the NuGet packages name and required version.
{
"frameworks": {
"net46": {
"dependencies": {
"Microsoft.Azure.KeyVault": "1.0.0",
"Microsoft.IdentityModel.Clients.ActiveDirectory": "2.14.201151115"
}
}
}
}
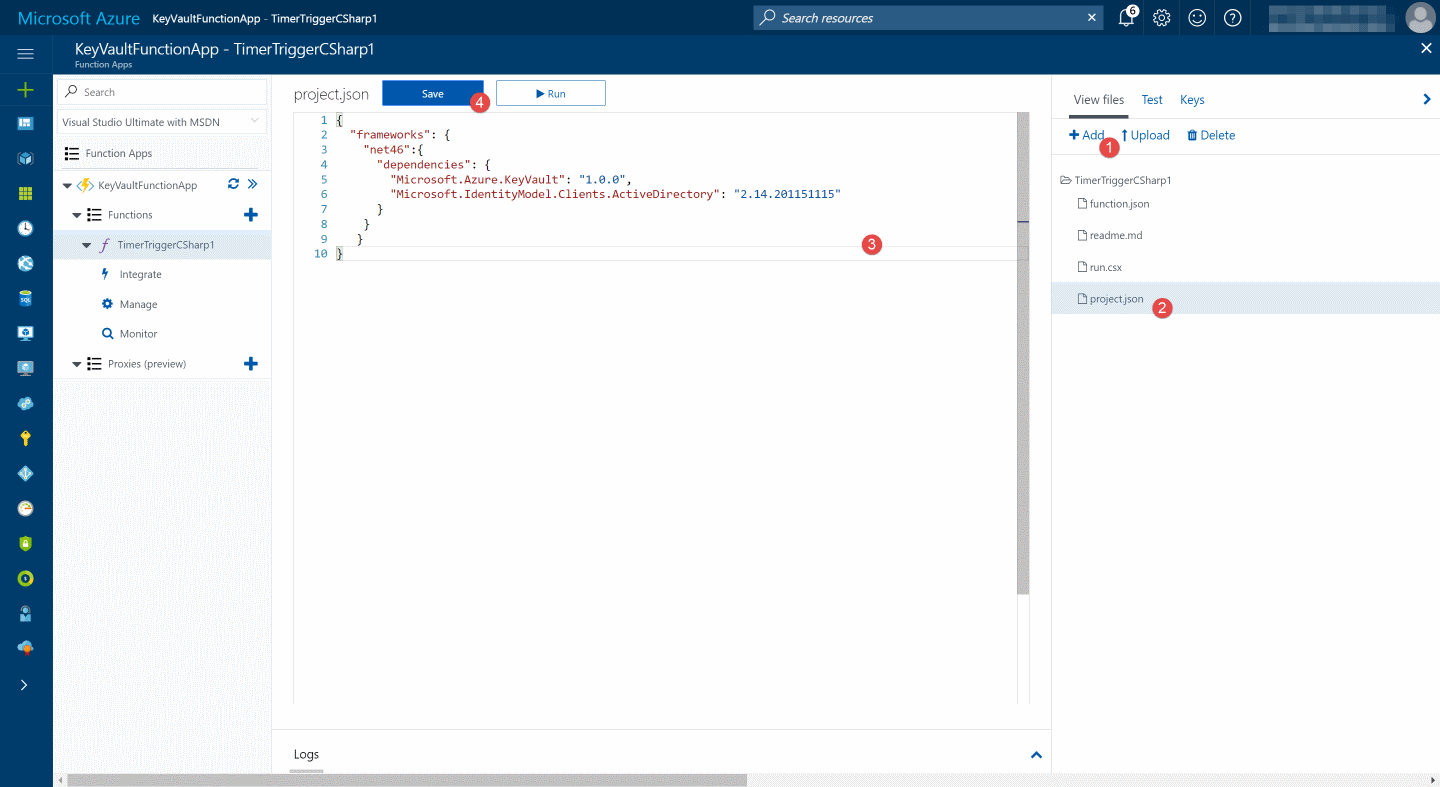
Executing the function, retrieves the secret details from the Key Vault and logs it as shown below.
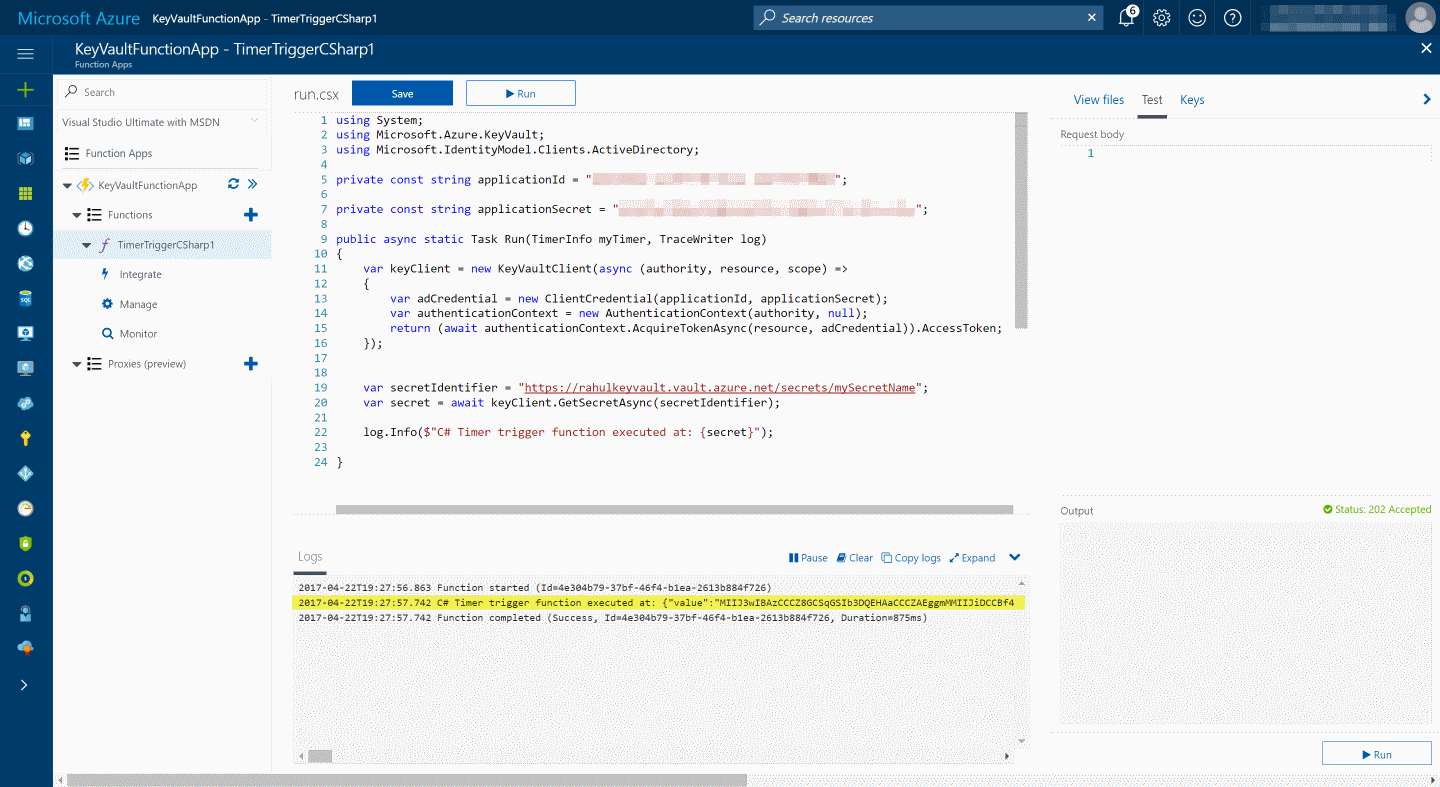
Hope this helps you to get started with Key Vault in Azure Functions and keep your sensitive data secure.
Rahul Nath Newsletter
Join the newsletter to receive the latest updates in your inbox.